c++11 改进设计模式 Singleton模式
关于学习 《深入应用c++11》的代码笔记:
c++11之前是这么实现的
template<typename T> class Singleton{ public: static T* Instance(){ if (m_pInstance == nullptr) m_pInstance = new T(); return m_pInstance; } template<typename T0> static T* Instance(T0 arg0){ if (m_pInstance == nullptr) m_pInstance = new T(arg0); return m_pInstance; } template<typename T0,typename T1> static T* Instance(T0 arg0, T1 arg1){ if (m_pInstance == nullptr) m_pInstance = new T(arg0, arg1); return m_pInstance; } template<typename T0, typename T1,typename T2> static T* Instance(T0 arg0, T1 arg1,T2 arg2){ if (m_pInstance == nullptr) m_pInstance = new T(arg0, arg1,arg2); return m_pInstance; } template<typename T0, typename T1, typename T2,typename T3> static T* Instance(T0 arg0, T1 arg1, T2 arg2,T3 arg3){ if (m_pInstance == nullptr) m_pInstance = new T(arg0, arg1, arg2,arg3); return m_pInstance; } template<typename T0, typename T1, typename T2, typename T3,typename T4> static T* Instance(T0 arg0, T1 arg1, T2 arg2, T3 arg3,T4 arg4){ if (m_pInstance == nullptr) m_pInstance = new T(arg0, arg1, arg2, arg3,arg4); return m_pInstance; } template<typename T0, typename T1, typename T2, typename T3, typename T4,typename T5> static T* Instance(T0 arg0, T1 arg1, T2 arg2, T3 arg3, T4 arg4,T5 arg5){ if (m_pInstance == nullptr) m_pInstance = new T(arg0, arg1, arg2, arg3, arg4,arg5); return m_pInstance; } static T* GetInstance() { if (m_pInstance == nullptr) throw std::logic_error("the instance is not init,please init the instance first"); return m_pInstance; } static void DestroyInstance(){ delete m_pInstance; m_pInstance = nullptr; } private: Singleton(void); virtual ~Singleton(void); Singleton(const Singleton&); Singleton& operator = (const Singleton); static T* m_pInstance; }; template<class T> T* Singleton<T>::m_pInstance = nullptr; //============================================ struct A{ A(){} }; struct B{ B(int x){} }; struct C{ C(int x, double y){} }; int _tmain(int argc, _TCHAR* argv[]) { Singleton<A>::Instance(); Singleton<A>::Instance(); Singleton<B>::Instance(1); Singleton<C>::Instance(1,3.14); Singleton<A>::DestroyInstance(); Singleton<B>::DestroyInstance(); Singleton<C>::DestroyInstance(); return 0; }
c++11之后可以简略一点,使用了可变模板参数
template<typename T> class Singleton{ public: template <typename... Args> static T* Instance(Args&&... args){ if (m_pInstance == nullptr) m_pInstance = new T(std::forward<Args>(args)...); return m_pInstance; } static T* GetInstance(){ if (m_pInstance == nullptr) throw std::logic_error("the instance is not init,please initialize the instance first"); return m_pInstance; } static void DestroyInstance() { delete m_pInstance; m_pInstance = nullptr; } private: Singleton(void); virtual ~Singleton(void); Singleton(const Singleton&); Singleton& operator=(const Singleton&); private: static T* m_pInstance; }; template<class T>T* Singleton<T>::m_pInstance = nullptr; #include <iostream> #include <string> using namespace std; struct A{ A(const string&){ cout << "lvalue" << endl; } A(string&&x){ cout << "rvalue" << endl; } }; struct B{ B(const string&){ cout << "lvalue" << endl; } B(string&& x){ cout << "rvalue" << endl; } }; struct C{ C(int x, double y){} void Fun(){ cout << "Test" << endl; } }; int _tmain(int argc, _TCHAR* argv[]) { string str = "bb"; Singleton<A>::Instance(str); Singleton<B>::Instance(std::move(str)); Singleton<C>::Instance(1,3.14); Singleton<C>::GetInstance()->Fun(); Singleton<A>::DestroyInstance(); Singleton<B>::DestroyInstance(); Singleton<C>::DestroyInstance(); return 0; }
作 者: itdef
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力
欢迎转帖 请保持文本完整并注明出处
技术博客 http://www.cnblogs.com/itdef/
B站算法视频题解
https://space.bilibili.com/18508846
qq 151435887
gitee https://gitee.com/def/
欢迎c c++ 算法爱好者 windows驱动爱好者 服务器程序员沟通交流
如果觉得不错,欢迎点赞,你的鼓励就是我的动力

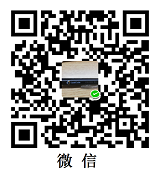